Fscale in Arduino
Finn Hammer, Tue Sept 27 2016, 07:42AM
Edit: Solved
It turnes out that fscale is not a proper function, but rather a script, where a whole lot of code is required at the bottom of the main code. Once added, the scaling works great.
.........................................
.......
I am a complete newbie in programming, but I am trying hard to learn.
I am building a custom lamp that takes input from 2 potentiometers, where first pot. blends from one 2000K led to another 4000K led, the other pot. dimms both leds.
The code works well enough, but the fading/blending over is not linear to the eye, as expected, so I have to add some sort of correction factor to the outputValue -erh, whatshammacallit?

I have tried to index an exponential array with the outputValue, and this works well, but the curve that the array expresses is not quite right, and producing the array seems cumbersome to me.
Therefore, I want to use the fscale function in Arduino

, but whenever I use it, I get either "fscale is not declared in this scope" or "fscale can not be used as a function" error messages.
Have you successfully used fscale in some code, then please help me along, since tweaking the "curve" parameter will probably make it easier to land on a satisfactory blend/fade of the leds.
Cheers, Finn Hammer,
In the code below:
I have commented out the map function of outputValueDimm, line 48, see picture below, and instead tried a line where I use fscale to do the same thing, but with non-linear mapping, and this results in the dreaded "fscale was not declared in this scope" error message
const int analogInPin0 = A0; // Analog input pin that the blend potentiometer is attached to
const int analogInPin1 = A1; // Analog input pin that the volume potentiometer is attached to
const int analogOutPin9 = 9; // Analog output pin that the LED 1 is attached to
const int analogOutPin6 = 6; // Analog output pin that the LED 1 is attached to
int sensorValue0 = 0; // value read from the pot @ analogInPin0 = A0
int sensorValue1 = 0; // value read from the pot @ analogInPin1 = A1
int outputValue = 0; // value output to the PWM (analog out)
int outputValue1 = 0; // value output to the PWM (analog out)
int outputValueDimm = 0; // value dimmimg factor
void setup() {
// initialize serial communications at 9600 bps:
Serial.begin(9600);
}
void loop() {
// read the analog in value:
sensorValue0 = analogRead(analogInPin0);
sensorValue1 = analogRead(analogInPin1); // map it to the range of the analog out:
outputValue = map(sensorValue0, 0, 1023, 0, 254);
outputValue1= map(sensorValue0, 0, 1023, 254, 0);
//below I have commented out a line with the "map" function, which works; and substituted it with a line that contains the fscale function, which does not work.
//outputValueDimm= map(sensorValue1, 0, 1023, 0, 254);
outputValueDimm= fscale(0, 1024, 0, 254, sensorValue0, 0)
analogWrite(analogOutPin9, outputValue*outputValueDimm/254);
analogWrite(analogOutPin6, outputValue1*outputValueDimm/254);
// print the results to the serial monitor:
Serial.print("sensor = ");
Serial.print(sensorValue1);
Serial.print("\t output = ");
Serial.println(outputValueDimm);
// wait 2 milliseconds before the next loop
// for the analog-to-digital converter to settle
// after the last reading:
delay(20);
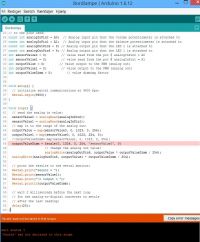
Re:
Fscale in Arduino
Blackcurrant, Tue Sept 27 2016, 03:12PM
I find this helpful when trying to remember C stuff
Re:
Fscale in Arduino
Finn Hammer, Tue Sept 27 2016, 04:05PM
All,
I guess that most people who has written code feel particularly good about the first piece they turned out.
Being an oldtimer myself, I feel exedingly good about having written this short piece, and it does the job!
Cheers, Finn Hammer
//Table lamp with variable colour temperature and volume. 27-09-2016 Finn Hammer
// This code reads the voltages on analog pins A0 and A1 and writes a PWM signal to D9 and D11.
// The voltages are generated by potentiometers.
// The PWM signals drive LED's directly or are used to drive power converters.
//fscale is used to create a logarithmic response between potentiometer and LED.
//This should make the fade look linear and keep an even output during blend from 2000K to 4000K
const int analogInPin0 = A0; // Analog input pin that the volume potentiometer is attached to
const int analogInPin1 = A1; // Analog input pin that the balance potentiometer is attached to
const int analogOutPin9 = 9; // Analog output pin that the LED 1 is attached to
const int analogOutPin6 = 6; // Analog output pin that the LED 2 is attached to
int sensorValue0; // value read from the pot @ analogInPin0 = A0 ->this blends the LED's
int sensorValue1; // value read from the pot @ analogInPin1 = A1 -> this fades the LED's
int outputValue2000K; // output to the PWM, LOG corrected with "fscale"
int outputValue4000K; // output to the PWM, LOG corrected with "fscale"
int outputValueDimm; // value dimmimg factor from dimming pot.
int fscaleBlendfactor = -3; //adjust this factor to obtain even output when blending from warm to cold white
int fscaleDimmfactor = -5; //adjust this for a linear dimming lamp
void setup() {
Serial.begin(9600);
}
void loop(){
//Read the "blend" pot.
sensorValue0 = analogRead(analogInPin0);
//change linear (blend) value to log. scale with "fscale" and produce mirrored image
outputValue2000K = fscale( 0, 1023, 0, 254, sensorValue0, fscaleBlendfactor);
outputValue4000K = fscale( 0, 1023, 254, 0, sensorValue0, fscaleBlendfactor);
//Read the "fade" pot.
sensorValue1 = analogRead(analogInPin1);
//change linear (fade) value to log. scale with "fscale"
outputValueDimm= fscale( 0, 1023, 0, 254, sensorValue1, fscaleDimmfactor);
// Combine values from blend pot. and dimm pot.
analogWrite(analogOutPin9, outputValue2000K * outputValueDimm / 254);
analogWrite(analogOutPin6, outputValue4000K * outputValueDimm / 254);
//print input and output values for debugging purposes.....

Serial.print("Blendpot = ");
Serial.print(sensorValue0);
Serial.print("\t 2K = ");
Serial.print(outputValue2000K);
Serial.print(" 4K = ");
Serial.print(outputValue4000K);
Serial.print("\t Dimmpot = ");
Serial.print(sensorValue1);
Serial.print(" Fade = ");
Serial.println(outputValueDimm);
}
//The rest below is the "fscale" code:
float fscale( float originalMin, float originalMax, float newBegin, float
newEnd, float inputValue, float curve){
float OriginalRange = 0;
float NewRange = 0;
float zeroRefCurVal = 0;
float normalizedCurVal = 0;
float rangedValue = 0;
boolean invFlag = 0;
// condition curve parameter
// limit range
if (curve > 10) curve = 10;
if (curve < -10) curve = -10;
curve = (curve * -.1) ; // - invert and scale - this seems more intuitive - postive numbers give more weight to high end on output
curve = pow(10, curve); // convert linear scale into lograthimic exponent for other pow function
// Check for out of range inputValues
if (inputValue < originalMin) {
inputValue = originalMin;
}
if (inputValue > originalMax) {
inputValue = originalMax;
}
// Zero Refference the values
OriginalRange = originalMax - originalMin;
if (newEnd > newBegin){
NewRange = newEnd - newBegin;
}
else
{
NewRange = newBegin - newEnd;
invFlag = 1;
}
zeroRefCurVal = inputValue - originalMin;
normalizedCurVal = zeroRefCurVal / OriginalRange; // normalize to 0 - 1 float
// Check for originalMin > originalMax - the math for all other cases i.e. negative numbers seems to work out fine
if (originalMin > originalMax ) {
return 0;
}
if (invFlag == 0){
rangedValue = (pow(normalizedCurVal, curve) * NewRange) + newBegin;
}
else // invert the ranges
{
rangedValue = newBegin - (pow(normalizedCurVal, curve) * NewRange);
}
return rangedValue;
;
}
Re:
Fscale in Arduino
Finn Hammer, Tue Sept 27 2016, 04:09PM
Blackcurrant wrote ...
I find this helpful when trying to remember C stuff
-And thanks a lot for that!