Rain Drop Terminal Velocity
Slava, Sun Aug 28 2016, 09:04PM

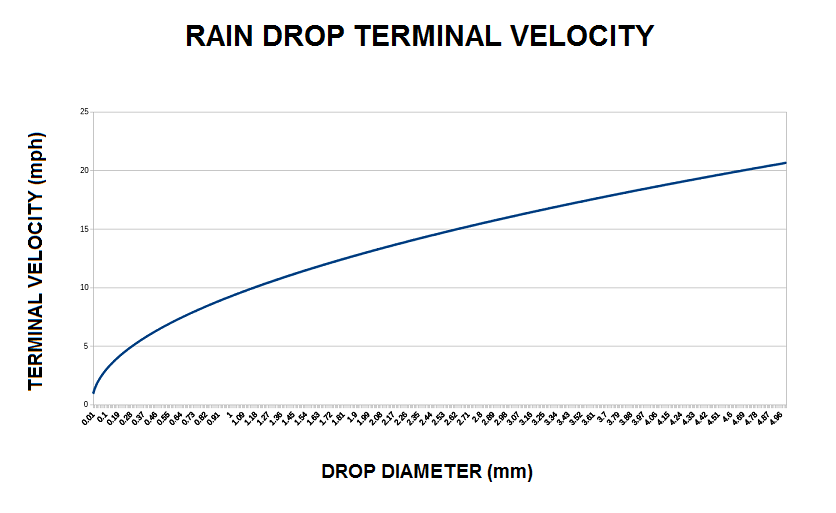
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace RainDrop001
{
class Program
{
static double Drag(double velocity, double diameter) // COMPUTE AIR DRAG
{
double area = Math.PI * Math.Pow(diameter / 2, 2);
//F = .5 * density_of_air * velocity^2 * drag_coef * area
return .5 * 1.225 * Math.Pow(velocity, 2) * .47 * area * Math.Sign(velocity);
}
static void Main(string[] args)
{
for (double d = 0.01; d < 5; d += .01)
{
double height = 500; // initial height
double diam = d * 0.001; // diameter of rain drop
double mass = ((4 / 3) * Math.PI * Math.Pow(diam / 2, 3)) * 1000;
double v = 0; // initial velocity
double dt = 0.0001; // delta time
while (height > 0)
{
double a = (-Drag(v, diam) / mass) - 9.8; // calculate acceleration
v += a * dt;
height += v * dt;
}
//File.AppendAllText(@"C:\Users\Rostislav\Desktop\raindrop.txt", d.ToString() + ", " + Math.Round(-v * 2.23694, 2).ToString() + Environment.NewLine);
Console.ForegroundColor = ConsoleColor.Green;
Console.WriteLine("DIAMETER (mm): " + d);
Console.WriteLine("SPEED (mph): " + Math.Round(v * 2.23694, 2));
Console.WriteLine("ENERGY (Joules): " + .5 * mass * Math.Pow(v,2));
Console.WriteLine();
}
Console.WriteLine("DONE.");
Console.ReadLine();
}
}
}