CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Thu Mar 29 2012, 01:09AM
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).Related Web Sources:Servo control using STM32VLDiscovery 
I have 2 ESC's and 2 servos, all require the normal servo PWM. the ESC's at 50 Hz PRF, the servos at 300HZ PRF.
code from the above link im trying to figure out:
+++++++++++++++++++++++++++++++++++++++++++++++++
+++
// STM32 4-channel Servo Demo for 24 MHz VL Discovery -
**link** #include "stm32F10x.h"
#include "STM32vldiscovery.h"
/
**************************************************
************************************/
void RCC_Configuration(void);
void GPIO_Configuration(void);
void NVIC_Configuration(void);
void TIM3_Configuration(void);
/
**************************************************
************************************/
volatile int servo_angle[4] = { 0, 0, 0, 0 }; // +/- 90 degrees, use floats for fractional
void TIM3_IRQHandler(void)
{
if (TIM_GetITStatus(TIM3, TIM_IT_Update) != RESET)
{
TIM_ClearITPendingBit(TIM3, TIM_IT_Update);
// minimum high of 600 us for -90 degrees, with +90 degrees at 2400 us, 10 us per degree
// timer timebase set to us units to simplify the configuration/math
TIM3->CCR1 = 600 + ((servo_angle[0] + 90) * 10); // where angle is an int -90 to +90 degrees, PC.6
TIM3->CCR2 = 600 + ((servo_angle[1] + 90) * 10); // where angle is an int -90 to +90 degrees, PC.7
TIM3->CCR3 = 600 + ((servo_angle[2] + 90) * 10); // where angle is an int -90 to +90 degrees, PC.8
TIM3->CCR4 = 600 + ((servo_angle[3] + 90) * 10); // where angle is an int -90 to +90 degrees, PC.9
}
}
/
**************************************************
************************************/
int main(void)
{
RCC_Configuration();
GPIO_Configuration();
NVIC_Configuration();
TIM3_Configuration();
while(1)
{
// Insert code here to stroke your servos
}
}
/
**************************************************
************************************/
void RCC_Configuration(void)
{
// clock for GPIO and AFIO (Remap)
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC | RCC_APB2Periph_AFIO, ENABLE);
// clock for TIM3
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM3, ENABLE);
}
/
**************************************************
************************************/
void NVIC_Configuration(void)
{
NVIC_InitTypeDef NVIC_InitStructure;
/* Configure the NVIC Preemption Priority Bits */
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_0);
/* Enable the TIM3 Interrupt */
NVIC_InitStructure.NVIC_IRQChannel = TIM3_IRQn;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 2;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
}
/
**************************************************
************************************/
void GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
// PC.06 TIM3_CH1
// PC.07 TIM3_CH2
// PC.08 TIM3_CH3
// PC.09 TIM3_CH4
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_6 | GPIO_Pin_7 | GPIO_Pin_8 | GPIO_Pin_9;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(GPIOC, &GPIO_InitStructure);
GPIO_PinRemapConfig(GPIO_FullRemap_TIM3, ENABLE); // Remap to get signal on PC.06-09
}
/
**************************************************
************************************/
void TIM3_Configuration(void)
{
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
TIM_OCInitTypeDef TIM_OCInitStructure;
// Simplify the math here so TIM_Pulse has 1 us units
// Use (24-1) for VL @ 24 MHz
// Use (72-1) for STM32 @ 72 MHz
TIM_TimeBaseStructure.TIM_Prescaler = 24 - 1; // 24 MHz / 24 = 1 MHz
TIM_TimeBaseStructure.TIM_Period = 20000 - 1; // 1 MHz / 20000 = 50 Hz (20 ms)
TIM_TimeBaseStructure.TIM_ClockDivision = TIM_CKD_DIV1;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInit(TIM3, &TIM_TimeBaseStructure);
// Set up 4 channel servo
TIM_OCInitStructure.TIM_OCMode = TIM_OCMode_PWM1;
TIM_OCInitStructure.TIM_OutputState = TIM_OutputState_Enable;
TIM_OCInitStructure.TIM_Pulse = 600 + 900; // 1500 us - Servo Top Centre
TIM_OCInitStructure.TIM_OCPolarity = TIM_OCPolarity_High;
TIM_OC1Init(TIM3, &TIM_OCInitStructure); // Channel 1 configuration = PC.06 TIM3_CH1
TIM_OC2Init(TIM3, &TIM_OCInitStructure); // Channel 2 configuration = PC.07 TIM3_CH2
TIM_OC3Init(TIM3, &TIM_OCInitStructure); // Channel 3 configuration = PC.08 TIM3_CH3
TIM_OC4Init(TIM3, &TIM_OCInitStructure); // Channel 4 configuration = PC.09 TIM3_CH4
// turning on TIM3 and PWM outputs
TIM_Cmd(TIM3, ENABLE);
TIM_CtrlPWMOutputs(TIM3, ENABLE);
// TIM IT enable
TIM_ITConfig(TIM3, TIM_IT_Update, ENABLE);
}
++++++++++++++++++++++++++++++++++++++++++++++++
+++++++++++++
more code shortly...
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Fri Mar 30 2012, 04:23AM
PID loops and Kalman filters...ok ive found the "PID without a PhD" PDF, and other sources:
Improving the Beginner’s PID
"PID Without A PhD" (Pdf)

feed forward and velocity, acceleration.
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Thu Apr 19 2012, 07:16AM
the Discovery boards:
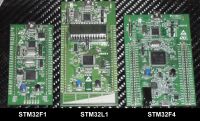
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Tue Jul 10 2012, 07:38PM
OK heres the list:
-- lets just talk about the pitch axis for simplicity, the other axis' will be parallel and similar anyway.
-- lets define the pitch axis as a "Y" axis, and nose up means positive above zero, while nose down means negative degrees below zero.
-- there are gyros in the kk board, but i dont have access to them or thier code.
-- i want to seperate navigation and stability, focusing only on stability for now. i want it to hover, well do more later
-- Ill keep my gyros 10 times slower than the kk's gyros, to avoid aliasing and or oscillating feedback.
-- ill post a schematic below of the control flow
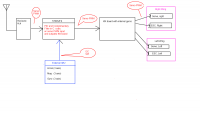
Re: CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
AndrewM, Tue Jul 10 2012, 08:34PM
I'm not going to look up the specs for all these board, why don't you make a simple post regarding your plan?
What is a KK board. Its expecting servo PWM input... so this must be some kind of off the shelf gyro?
If that board isn't controlling your stability adequate... why are you using it? I'm not sure I follow the logic of having a micro running a PID controller... feeding into an off the shelf PID controller.
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Tue Jul 10 2012, 08:39PM
AndrewM wrote ...
I'm not going to look up the specs for all these board, why don't you make a simple post regarding your plan?
i wasnt expecting anyone to do so.
AndrewM wrote ...
What is a KK board. Its expecting servo PWM input... so this must be some kind of off the shelf gyro?
If that board isn't controlling your stability adequate... why are you using it? I'm not sure I follow the logic of having a micro running a PID controller... feeding into an off the shelf PID controller.
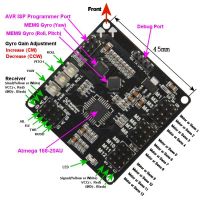
well it doesnt seem to be worth a dam, we can get rid of it. all it does is take in a RC control from the human, pass it to a gyro stability program and spilt up that result to the serovs and fans. Minsoo kim didnt put accelerometers on it so it cant hold it self level. for 240-350$ more i guess i could buy his lame upgrade, the black board which im using know cost me 140$ already.
what id like to do, since he won t answer my emails, is just to release an open source code here at 4hv that anyone could load on an STM32 board for $9-$25 then go fly. then yould need some spark fun orsimilar supplier of the imu, but still its cheaper and better.
Re: CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
AndrewM, Tue Jul 10 2012, 09:31PM
Yeah thats what I thought. I have a similar unit for fixed wing aircraft and it works pretty well... but I'd be pretty wary about dropping it into something like this. From your videos its just not up to snuff so I don't think adding another PID layer upstream is going to be a good solution.
So you basic sensor suite is... 3 axis gyro and 3 axis accel? And all of this is accessible by your STM32?
I think your basic case should be a stable hover... that is, all rates are zero and the angle of the gravity vector equals your user's commanded angle (ie. the stick position). You said in your other thread you want to control Pitch first (indeed I think that will be your toughest axis to control). So lets call pitch "θ"...
Proportional error:
E_p = θ_dot_gyro
Integral error:
E_i = θ_actual - θ_commanded
The derivative term is harder since you don't have a derivative sensor. You'll want to make sure you have nice clean data coming out of your rate sensor, otherwise your derivative terms can go nuts; if you can't clean the data then you'll want to implement a running average or something, depending on your sample rate.
To be honest I'd try PI control first... because I bet that even if you DO implement D control that it will be so noisy you'll need to turn the gain down to uselessness.
Then your loop output would be:
u = Ki * E_i + Kp * E_p
You'll need to write your equations of motion in order to determine how to use the u signal... but almost surely you'll want u to signal the motion of your fan angle servos together (ie. left and right side equally).
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Wed Jul 11 2012, 12:11AM
AndrewM wrote ...
Yeah thats what I thought. I have a similar unit for fixed wing aircraft and it works pretty well... but I'd be pretty wary about dropping it into something like this. From your videos its just not up to snuff so I don't think adding another PID layer upstream is going to be a good solution.
yep we'll ditch the KKmulticopter board, and move on.
AndrewM wrote ...
So you basic sensor suite is... 3 axis gyro and 3 axis accel? And all of this is accessible by your STM32?
yep, i can access the gyro at 400 or 800 hz, and the accel at 400 to 2000 dps... this imu is fully under my control and coded right to the STM32.
AndrewM wrote ...
I think your basic case should be a stable hover... that is, all rates are zero and the angle of the gravity vector equals your user's commanded angle (ie. the stick position). You said in your other thread you want to control Pitch first (indeed I think that will be your toughest axis to control). So lets call pitch "θ"...
Proportional error:
E_p = θ_dot_gyro
Integral error:
E_i = θ_actual - θ_commanded
ok, my brain is slowing...
AndrewM wrote ...
The derivative term is harder since you don't have a derivative sensor. You'll want to make sure you have nice clean data coming out of your rate sensor, otherwise your derivative terms can go nuts; if you can't clean the data then you'll want to implement a running average or something, depending on your sample rate.
To be honest I'd try PI control first... because I bet that even if you DO implement D control that it will be so noisy you'll need to turn the gain down to uselessness.
ok, well we can leave out the D term for now, but i was going to use a complimentary filter as suggested by big5824, to "de-noise" the signal.
big5824's site :

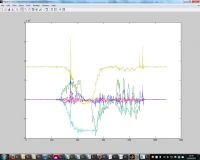
raw data
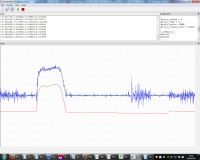
filtered with complimetary scheme
AndrewM wrote ...
Then your loop output would be:
u = Ki * E_i + Kp * E_p
You'll need to write your equations of motion in order to determine how to use the u signal... but almost surely you'll want u to signal the motion of your fan angle servos together (ie. left and right side equally).
ok i see the above as a simple PI type deal, i can figure out how to code that, but what do you mean by
"You'll need to write your equations of motion ..."
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
AndrewM, Wed Jul 11 2012, 12:40AM
Patrick wrote ...
ok i see the above as a simple PI type deal, i can figure out how to code that, but what do you mean by "You'll need to write your equations of motion ..."
Write equations for the pitch rate, θ_dot, in terms of the fan state.
For a PI controller it'll be simple, like:
θ_dot = F_fan * distance_to_c.m. / I_pitch
distance_to_c.m. will be a function of the fan servo angle... when you write that equation you should see immediately why steve and I told you to get your fans as far above the center of mass as possible.
When you have that equation you can model the system and simulate how to utilize the output of the loop, u.
Eventually when you implement a more complex controller you can add things to the EOM, like the acceleration of the fan servos; changing the fan angle will jerk the body of your craft around, for example. You can make the EOMs as complex as you like.
Also: keep in mind that you'll need a solution to correct your gyro drift - this is apparently where people have implemented kalman filters and the like. I haven't ever built a control loop like this so I don't know if its a necessity or a "nice-to-have." Seems to me that, as a first order attempt, a simple high pass filter on the gyro output before the controller should be enough to get things working...
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Wed Jul 11 2012, 12:56AM
AndrewM wrote ...
Patrick wrote ...
ok i see the above as a simple PI type deal, i can figure out how to code that, but what do you mean by "You'll need to write your equations of motion ..."
Write equations for the pitch rate, θ_dot, in terms of the fan state.
For a PI controller it'll be simple, like:
θ_dot = F_fan * distance_to_c.m. / I_pitch
distance_to_c.m. will be a function of the fan servo angle... when you write that equation you should see immediately why steve and I told you to get your fans as far above the center of mass as possible.
When you have that equation you can model the system and simulate how to utilize the output of the loop, u.
Eventually when you implement a more complex controller you can add things to the EOM, like the acceleration of the fan servos; changing the fan angle will jerk the body of your craft around, for example. You can make the EOMs as complex as you like.
Also: keep in mind that you'll need a solution to correct your gyro drift - this is apparently where people have implemented kalman filters and the like. I haven't ever built a control loop like this so I don't know if its a necessity or a "nice-to-have." Seems to me that, as a first order attempt, a simple high pass filter on the gyro output before the controller should be enough to get things working...
ok i see what youre getting at with the equation now.
are you meaning dot product for theta_dot?
F = newtons
dist = meters
what units are I_pitch? is that an angle?
a projection of one onto the other?
Re: CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
AndrewM, Wed Jul 11 2012, 01:17AM
In controls (maybe just aeronautics?) "dot" is the same as prime which is the same as derivative.
So theta_dot = d theta/dt (angular velocity)
theta_dotdot = d2 theta/ dt2 (angular acceleration)
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Wed Jul 11 2012, 01:44AM
AndrewM wrote ...
In controls (maybe just aeronautics?) "dot" is the same as prime which is the same as derivative.
So theta_dot = d theta/dt (angular velocity)
theta_dotdot = d2 theta/ dt2 (angular acceleration)
whoops! i see what you meant, yes i knew that, brain lapse... im not good at ASCII math anyway. do you know anything about programming in C?
ok so
theta dot= (d theta )/( dt) which effectivley means:
(change in angle theta) / (change in time)therefore if the time interval is consecutive and constant:
(d theta)/(dt) = (current theta - previous theta)/(time interval)and i think thats what Brett Beaureguard means in this line :
[ double dErr = (error - lastErr) / timeChange; ]
Re: CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
AndrewM, Wed Jul 11 2012, 04:22AM
I've never been very good at reading other people's code, I usually just write my own... so I didn't read that page.
But yeah, looks like numerical differentiation to me.
Re: CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Wed Jul 11 2012, 05:10AM
well you and i are going to get this working so well need to figure out rolling, pitch collective and yaw and pid's and every other little thing we need, i want this done in less than one year from now.
I really appreciate your help, im going to stay on this everyday.
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Carbon_Rod, Wed Jul 11 2012, 08:53AM
Typically for UAV aerial acrobatics, people use Minimum Jerk Trajectory planning with Visual Servoing.
If you start to run out of time, openservo is a cheap mod that will reduce some of the errors that you are about to encounter...

Note that most gyros & some accelerometers will have oversampling error reduction, and low pass filter options...
i.e. simply use the on chip noise filters if you know the craft will not exceed a few g or high angular rates.
@AndrewM There are few robust alternatives outside the consensus of prior threads...
... the current approach will unlikely stay in tune very long...


Note a 200gram 1GHz wifi enabled mini computer is $68 delivered....

And it runs a popular distro:


Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Wed Jul 11 2012, 02:26PM
Carbon_Rod wrote ...
Note that most gyros & some accelerometers will have oversampling error reduction, and low pass filter options...
i.e. simply use the on chip noise filters if you know the craft will not exceed a few g or high angular rates.
@AndrewM There are few robust alternatives outside the consensus of prior threads...
... the current approach will unlikely stay in tune very long...


Note a 200gram 1GHz wifi enabled mini computer is $68 delivered....

And it runs a popular distro:


i will use the onboard low pass filter that my chips already supportand i still need to worry about gyro drift, but as for your 68$ computer thats not a real embedded system, and i cant configure pins on it or a RTOS like i can with a pic, ardiuno, STAMP or STM32.
edit, super dooper important: ]filter.pdf[/file]
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Carbon_Rod, Thu Jul 12 2012, 05:04AM
In general, latency tolerant functions are located within an RTlinux kernel based OS with usb host stack and wifi.
Most RTOSs are not what the name implies, as the last real RTOS was MSDOS.

In general, people who believe otherwise are better off using multiple chips/boards....
A 12Mbps USB host link will add little latency to consumer sensors given maximum sample rates will rarely exceed 400Hz...
Note a 1.5GHz Cortex-A8 A10 with 1GB ram and a cheap interface chip for i/o is flexible...

Re: CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Thu Jul 12 2012, 05:50AM
I dispute your claims, yet that clip was funny.
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Carbon_Rod, Thu Jul 12 2012, 08:40AM
Specifically, schedulers need to save multiple machine states for threading/message-passing, interrupts may overlap ISRs to randomize stack-pointer/register save times, and multi-core/GPU systems have several types of resource contention.
Most multitasking RTOS are approximating a fixed latency scheduler even if written in Assembly. If the accumulated errors from timing jitter are negligible/profiled than people sell it as an "RTOS".

"dispute" infers there is some inherent value in deception requiring negotiation...



Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Thu Jul 12 2012, 12:11PM
dispute the meaning of dispute all you want

Ill stick with my STM32's for the time being since they handle GPIO, I2C and SPI nativley, and a traditional computer doesnt.
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Carbon_Rod, Fri Jul 13 2012, 06:06AM
The xIMU AHRS algorithm (note the examples and pdfs):

Has been ported to the STM32

NI also has some samples of the Quaternion form for sparkfun's IMU

The freeIMU project has been ported to several boards

Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Tue Nov 06 2012, 03:48AM
I would appreciate any help in modding or finding existing code fro SLAM , i need it to be in C, ive heard of and seen a type of "tinySLAM" but ive been told it runs on linux or windows PCs only... Is this true? I really need a STM32 capable slam model.
i continue to look on my own.
I have found this:

it looks much more capable than what i need.
Re: CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Pinky's Brain, Tue Nov 06 2012, 05:05AM
It has some filesystem code to write images of the maps it constructs, but apart from that there are no major dependencies which would make it hard to convert it to run on a microcontroller.
Performance might be an issue, if it's designed for *nix it's probably designed for embedded computer boards like the beaglebone more than the STM32. I haven't tried to follow the algorithm though.
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Carbon_Rod, Tue Nov 06 2012, 05:16AM
Recall the map data size, limited mcu RAM, and i/o speed of the disk..
The URG is overpriced, but can offer cm accuracy on some models (PM for tips).
...The oak stood strong in its resolve...

ROS is a mature system that relies on this library to do part of the heavy lifting.

It works well on linux, has 95% of the features you don't know you need yet, and offers built-in drivers for the unit you mentioned.
Cheers,
Rod
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Tue Nov 06 2012, 05:32AM
Carbon_Rod wrote ...
Recall the map data size, limited mcu RAM, and i/o speed of the disk..
The URG is overpriced, but can offer cm accuracy on some models (PM for tips).
...The oak stood strong in its resolve...

ROS is a mature system that relies on this library to do part of the heavy lifting.

It works well on linux, has 95% of the features you don't know you need yet, and offers built-in drivers for the unit you mentioned.
Cheers,
Rod
im sure that oak comment was a slam at my wrong headediness...
Im not using the hykuyo devices for cost and wieght reasons...
Im not ignoring your advice Carbon Rod and i appreciate the scare tactics
(if i win it will be becuase you, andrewM and steve mconnor have scared me to death 900 times.) but i have seen the WiFi and the desktops fail to fucntion so many times in 2011 and 2012 and these great 20k$ bots cant even make it through the window... i really need a embedded SLAM.
what SLAM features do you think ill need, yet dont realize yet? rememeber the course is a simple hallway with rooms and doorways, its not random or obsticle laden... Would it help it i drew the cousre out?
EDIT/UPDATE: found this for 55 USD, 25 Grams!!!

i just dont know how to interface to it !?
explanation between RPi and MK802II

Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Carbon_Rod, Tue Nov 06 2012, 06:17AM
It will depend on your selected sensor, but some methods are computationally feasible on low end systems.

It is already known best practices separate path-planning/mapping (latency tolerant commands), localized guidance (prevents bouncing into walls/floor/sky), and sensor telemetry filtering (bandwidth reduction).
A $12 wifi plug weighs 2.17g, and in theory can add extensive resources with a fixed communication cost.

Force "n" mode on your router, and most signal interference issues will likely subside.
Re: CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Tue Nov 06 2012, 06:35AM
the N wireless router were used, its complicated settings and code that are cuasing the failures not the transmitting/recieving router itself...
in august 2012 it seemed the routers stayed up and running almost constantly, but the machines/desktops just wouldnt syncronize for useful data. the machines that were flying often hovered, then with a 3 second delay flew themselves into walls then, while crashed in pieces on the floor, it then tried to execute a collision avoiding turn.
Re: CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Carbon_Rod, Tue Nov 06 2012, 07:21AM
There are only 11 wifi channels on north American routers...
Hint: your system can use Japanese driver bands to operate outside a local crowded spectrum.
...fixed channel, and full WPA2 links are recommended.
Note sure if the RTL8188 can enter master mode, but it could act as the router if supported.
Re: CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Tue Nov 06 2012, 05:14PM
i just think including a WiFi router as a functionally required part, taunts failure in the face. If i use the Rpi, then theres no need for the router.
Re: CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Pinky's Brain, Wed Nov 07 2012, 08:14PM
You can get FPV antenna trackers for a little over 100$ BTW. Since in the US you are actually allowed to increase EIRP with high gain antennas this you should be able out drown out interference with that.
Re: CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Wed Nov 07 2012, 09:32PM
its not transmitting /recieving thats the problem, i think its complicated programmimg, missing real time slots of needed data.
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Pinky's Brain, Fri Nov 09 2012, 08:54AM
Patrick wrote ...
the N wireless router were used, its complicated settings and code that are cuasing the failures not the transmitting/recieving router itself...
in august 2012 it seemed the routers stayed up and running almost constantly, but the machines/desktops just wouldnt syncronize for useful data. the machines that were flying often hovered, then with a 3 second delay flew themselves into walls then, while crashed in pieces on the floor, it then tried to execute a collision avoiding turn.
With all due respect, if you can't do a simple remote procedure call over a reliable wireless connection of negligible latency and relatively high bandwidth you're doing it wrong. It's only complicated if you work hard at making it complicated.
That said, how are you so sure the connection was reliable? WLAN is prone to interference because it's such a polite protocol and sticks to a single channel, the delay could just as easily be from exponential back off and if they are stupid enough to use TCP that would only make it worse.
A nRF24L01+ based link might be more reliable.
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Carbon_Rod, Fri Nov 09 2012, 09:52AM
"Tunnels TCP over UDP packets. Runs in user space on Linux, Solaris, Cygwin (with IPv6 extension), and native Win32."

This method maintains a data stream over a stateless protocol:

The other tips discussed will mean rapid recovery from signal collisions, corruption, and throttling.
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Steve Conner, Fri Nov 09 2012, 10:11AM
There's no such thing as a simple remote procedure call. Distributed computing is always going to be tricky, and real-time deadlines only make things trickier.
I say this having written my own RPC library that can do 10,000 RPCs per second.

Re: CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Pinky's Brain, Fri Nov 09 2012, 02:33PM
Most of those problems come into existence when you don't treat the connection (and the remote party) as 100% reliable ... if as Patrick maintains they are that simplifies everything.
Although as I indicated, I kinda doubt the connection is that good.
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Steve Conner, Fri Nov 09 2012, 03:11PM
That's exactly the issue with distributed computing. When you call a regular function, you know it's going to execute and return a value. But when you do a remote procedure call, it could fail. Either it times out, or hangs forever, or if you decided to be very clever and do non-blocking transactions, your program already carried on without knowing if the RPC failed.
If you try to handle this gracefully, you get screwed by the complexity. If you ignore the issue and pretend that remote procedure calls will never fail, you get screwed when one eventually does fail.
I dealt with the issue by using a very simple RPC protocol and making sure the underlying hardware bus really was reliable. Patrick may need some other approach. I would have the drone default to hovering motionless, sending sensor data back to the desktop machine, until the desktop commanded it to make a move, along the lines of "Go 10 yards northeast and wait for my next command".
The drone could have a very basic collision detect algorithm on board to stop it from smashing directly into walls if given bad commands. It's easy to stop the commands getting corrupted in transit by just adding a CRC. But it's much harder to stop the desktop software from interpreting the sensor data wrongly and commanding the vehicle to fly into a wall that it didn't realise was there, so I would want a second opinion from a simple algorithm that has access to fast, accurate real-time data. Not "wall 10 yards away" but "wall real close right now!"
For extra brownie points, you could hook the desktop software up to a simulated vehicle in a virtual maze and test it without trashing anything, except a lot of 1s and 0s, but those are pretty cheap.

What does the AR Drone do when it loses its wi-fi connection?
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Fri Nov 09 2012, 06:12PM
Steve Conner wrote ...
What does the AR Drone do when it loses its wi-fi connection?
the Indian university teams found, if they unplug the wifi router, there Ar just hovers indefinatley, they had to activly transmit a fail-safe turn off command, so they were disqualified and prevented from flying at all in 2012.
Re: CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Steve Conner, Fri Nov 09 2012, 07:00PM
Is that a safety rule, that it has to shut down if it loses signal?
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Fri Nov 09 2012, 08:58PM
Steve Conner wrote ...
Is that a safety rule, that it has to shut down if it loses signal?
in the past its been complicated though.
yes there must be a positive safety fail... the absence of a "keep alive" signal must cuase shutdown.
They used to have nitro/alchol 700 size helis, a common kill mechanism was to have a servo pinch the fuel tube shut. however when one team did this back in 93ish, the engine ran lean off the float carb remanants producing more power, then it flew out of control closely past the epcot dome continued above the parking lot and crashed in front of a bunch of African school children. no one was injured, but after that they really have been on us about safety.
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Pinky's Brain, Fri Nov 09 2012, 08:59PM
Patrick wrote ...
EDIT/UPDATE: found this for 55 USD, 25 Grams!!!

i just dont know how to interface to it !?
The processor is nice, but with no GPIO breakout it's kinda limited ... the amount of GPIO broken out on Raspberry PI is sadly pathetic too.
The Cubieboard is shaping up to be the best platform for A10 hacking :

Dunno when it's shipping again though. In the meantime the Beaglebone is one of the nicest development boards with a relatively powerful processor, only 36 grams too.
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Fri Nov 09 2012, 09:04PM
Pinky's Brain wrote ...
The Cubieboard is shaping up to be the best platform for A10 hacking :

Dunno when it's shipping again though. In the meantime the Beaglebone is one of the nicest development boards with a relatively powerful processor, only 36 grams too.
will either of these run a slam model?
Re: CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Pinky's Brain, Fri Nov 09 2012, 09:44PM
Dunno, no idea how much processing powers they tend to take ... I think GHz range microprocessors with a GB of memory should be able to handle it, but dunno.
One problem with the beaglebone is that it doesn't come with a camera interface.
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Fri Nov 09 2012, 10:14PM
Pinky's Brain wrote ...
One problem with the beaglebone is that it doesn't come with a camera interface.
i have all that handled already (Classified, Most Secret)
i just need onboard, no communication SLAM and MDP.
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Sun Nov 11 2012, 03:25AM
BeagleBoard appears to be the solution. ->

, just 37 grams.
Since it runs multiple versions of Linux, then the SLAM versions that are linux friendly, should be able to be compiled for this system, right?
Re: CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Steve Conner, Sun Nov 11 2012, 08:08AM
I guess, but you might want to check that your chosen Linux distro supports your processor's FPU. The Raspberry Pi's Debian Wheezy just got it recently: older versions ran emulated floating point while the hardware FPU sat around doing nothing.
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Carbon_Rod, Tue Nov 13 2012, 07:59AM
The Rasberry Pi custom SSH access only kernel from Lady Ada has many of the drivers built in:

It only took a few hours to figure out the drivers and power supplies to support:
* i2c 9 DOF IMU and Altimeter/Thermometer board (ITG3205 BMA180 HMC5883L BMP085)
-postage stamp sized PCB unit placed well away from other boards
-i2c bus id flips depending on which release of the Pi used
* DHT22 (kind of intermittent at 3.3v)
* UART to Fastrax 501 GPS
-standard gpsd daemon works after removing com terminal from initab and boot params.
* 6 out of the 8 servo GPIO direct drives work if all bus ports active
-need to map around other io ports as the ko driver zeros GPIO on insmod
-can't use the analog-audio-output jack's PWM while active (it uses the DMA IRQ)
-must run "sudo rpi-update" to pull a kernel that can read this ko
I can confirm all the above peripherals are functional on Pi Model B r1 (DHT22 is a little flaky), and do not require any external parts besides the 3A 5v (Pi), 1A 3.3v LDO (sensor), and 1.5A 5v (servo test had no BEC from an ESC) regulated power supplies.
Next week:
* The $7 ebay rtl8188/rtl8192 USB micro wifi dongle will be tested soon
* SPI port, USB, and on-board 4MP camera port to be released soon
* OEM thermal-throttled-overclocking support (doubles performance in some cases)
* udptunnel is already in this armhf repository
Took 6 hours... mostly on google finding the servo/ESC drivers mknod bug fix.
Ada's Custom kernel repo has a few bugs in the wifi build...
Mostly their group just aggregates all the fringe driver projects in this kernel.

@Patrick
The over-sized system battery will chew though your weight budget faster than a few temporary "extra" bits on a PCB.
Most ROS modules are not necessary, but RPC services should work just fine.

Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Wed Nov 14 2012, 04:29AM
Carbon_Rod wrote ...
@Patrick
The over-sized system battery will chew though your weight budget faster than a few temporary "extra" bits on a PCB.
not sure what you mean here? are you reccomending the Rpi?
Re: CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Carbon_Rod, Wed Nov 14 2012, 05:27AM
So far, the Pi has proven trivial to integrate into a fixed wing autopilot setup (used to run on a GumStix).
The only problem I encountered was no one reminding people to check the verified hardware compatibility list for a 16GB SanDisk SDHC card etc. Just like a camera... not all cards work, and eBay is rife with con artists relabeling 2GB cards.
The battery's drain will impact flight times less if the processor intensive data interpretation is done on a ground station.
Re: CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Thu Nov 15 2012, 02:36AM
ok Carbon Rod, what SLAM features would you say are first essential, and next desirable? is there a SLAM that runs on Rpi you know of?
Re:
CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Carbon_Rod, Thu Nov 15 2012, 04:03AM
Your lidar thread suggests a plan to implement a solution for the bidirectional reflectance distribution function:

These cost $1700+ as they offer cm accuracy up to 6m, have minimal position noise, and relatively low data scan rates. The Pitch/Roll corrected lidar driver + 2D slam is already integrated in the discussed software packages.
Given the weight of 3D cameras like a Kinect, I am left to wonder if a practical economic alternative is available. Luckily, a fixed wing craft at 500M+ does not need to worry about walls.
Re: CSU Chico Tilt Rotor Flying Machine, (Programming and CPU, 3 of 3).
Patrick, Thu Nov 15 2012, 06:03AM
what about edge, wall, corner, waypoints and orientation? are there terms and methodoligies for all this or shouldijust start randomly coding?
i probally should have said this earlier, but i only need 2D SLAM... elevation isnt relevant.